Using React with a Python Backend
For this tutorial, we provide a template repository to show how to develop a single page application with react and a python backend.
For this tutorial, we provide a template repository to show how to develop a single page application with react and a python backend.
2 template repositories are provided: one using normal javascript
and a second using typescript
Both provide a very similar experience with getting your test application running.
What these repositories provide
These respositories provide an easy way to:
- install javascript and python packages to get started
- automatically start the python server using
yarn test
, which starts the python server, runs the jest tests and shuts down the python server automatically yarn start-sanic-test
starts the python server (based on sanic) with CORS permissions to prevent requests from your react application from being rejected (note, do not run the test version of the server in a production environment)
3a. we also fix bugs we've found when doingyarn start
when making live code edits, resulting in re-render errors.
3b. configuration to use proxies for bothjest
tests and the react SPA to communicate with the python server- Sample code:
4a. how ajest
test communicates with the python server
4b. example of the home page making a fetch to the server and rendering the response to the webpage
Prerequisites
For this sample code to work, we recommend the following to be pre-installed on your machine:
- Nodejs (this was tested using Node 18)
- yarn (yarn 3 was used, but older versions of yarn should be ok)
- python version 3.6+ (tested on python 3.10)
- virtualenv (used to create virtual environments)
Installation
For installation of the javascript workspace from here.
Installation can be done with the following commands:
git clone https://github.com/spherex-dev/react-app-python-template
cd react-app-python-template
./setup.sh
For installation of the typescript workspace from here
Installation can be done with the following commands:
git clone https://github.com/spherex-dev/react-app-ts-python-template
cd react-app-ts-python-template
./setup.sh
If you develop in vscode, installation of additional packages to fix linter errors can be done with yarn dlx @yarnpkg/sdks vscode
. When a dialogue in vscode pops up to use these libraries, click on yes
.
An example of the javascript workspace installation is provided below:
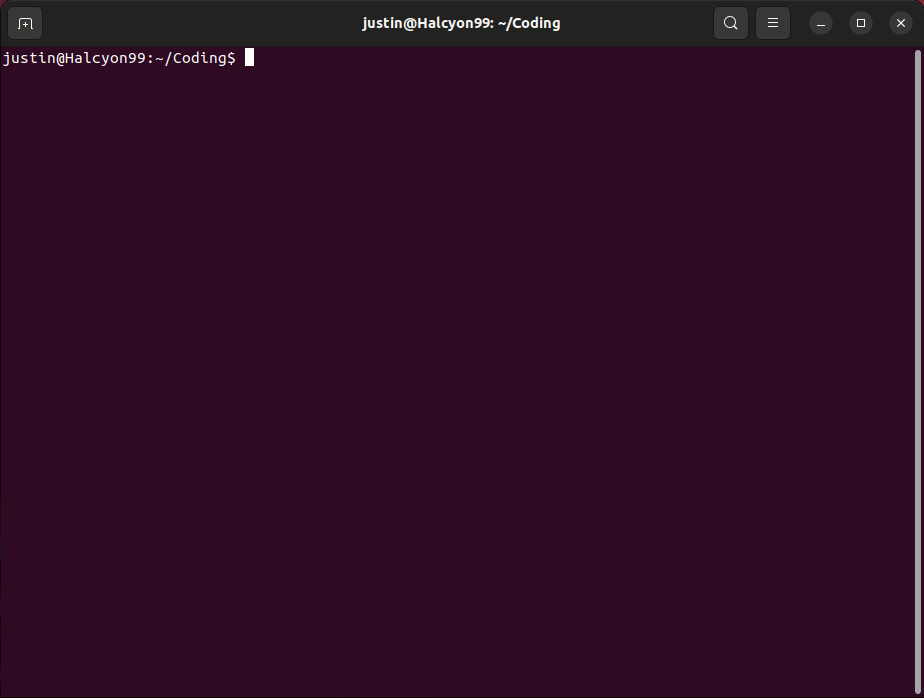
You'll see here that javascript and python packages are installed.
Running Jest Tests
Jest tests are simply run with jest test
to run all tests, you'll just need to hit a
once the prompt come up to run all tests
some fetch tests will run against the backend showing that data is being fetched.
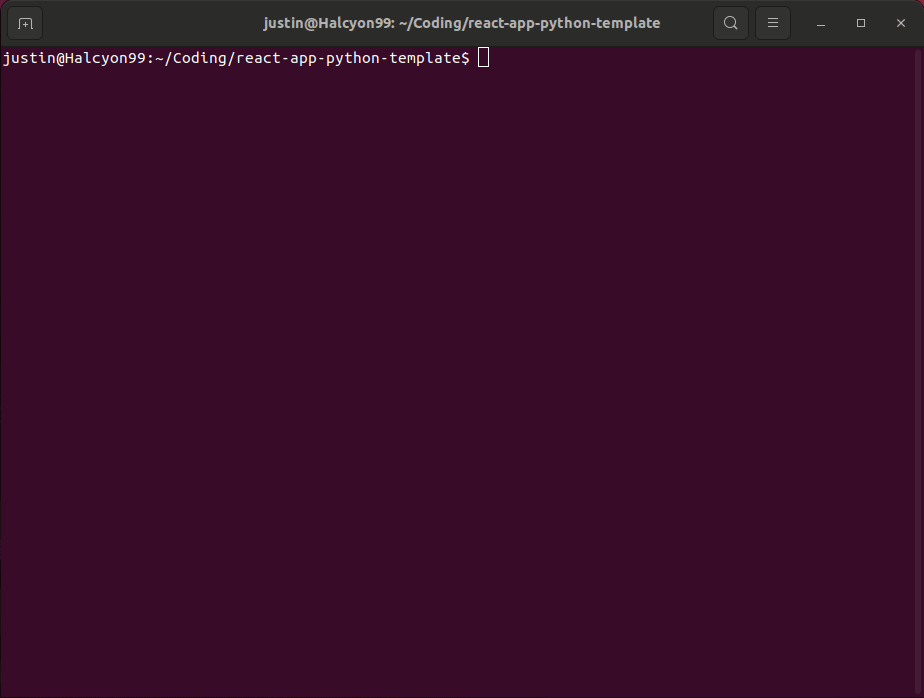
Running the Single Page Application
Running the single page application is done by running 2 commands:
yarn start-sanic-dev
which starts the python backendyarn start
which starts the single page application
The animated image below shows the react application starting and that a fetch and render occurs via the backend with "Greeting from backend is world
".
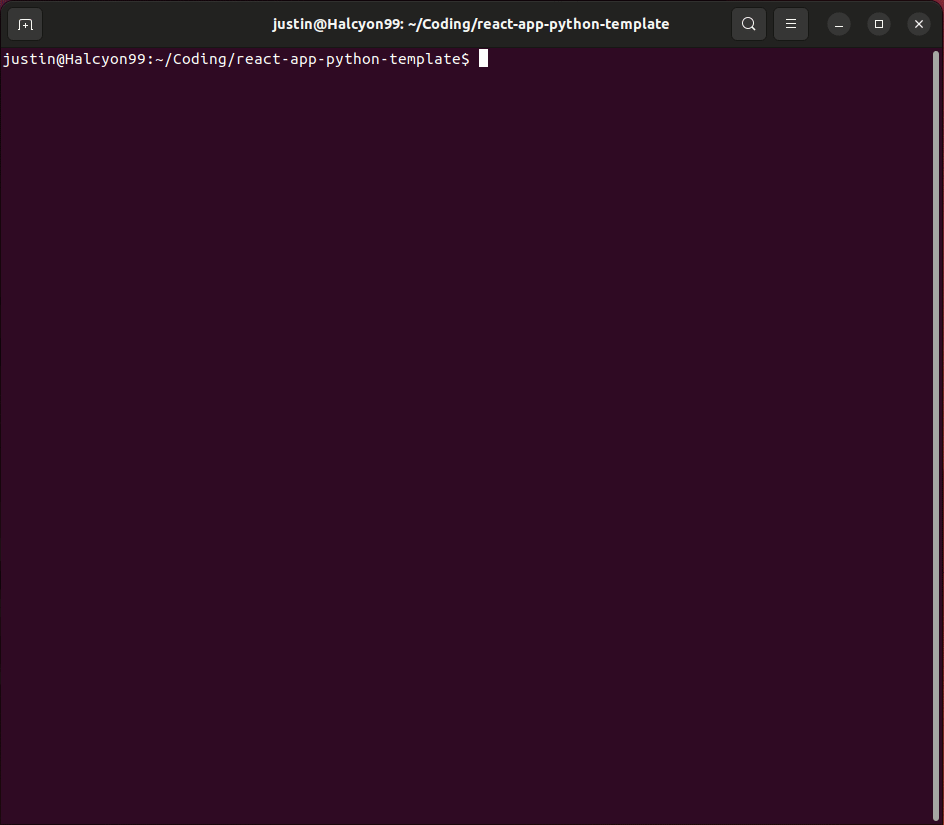
What is Sanic and how to add additional end points
Sanic is a http server, very similar to flask and was first at the time to implement async which was added in python 3.5. The syntax is nearly identitcal to flask and users with a background in flask should have no issues with using it. If you wish to use an alternative backend, feel free to make the replacement – just make sure that the backend server is able to requests from other origins when doing development.
Additional endpoints can be added via adding routes. A short description is provided here about routes. Routes can be added by either blueprints or the sanic app itself. Code for the blueprints are stored in backend/bp/*
. Blueprints are dynamically searched and added to the application so long as the files are saved with the following naming convention backend/bp/<blueprint_name>/views.py
. You can also add additional routes to backend/bp/hello/views.py
and create additional utility functions in src/utils/hello.js
to fetch data from the backend.
In addition, tests can be added in src/utils/hello.test.js
to run with yarn test
which runs jest
to perform unit tests. This especially nice as you will be able to test that functions are able to appropriately fetch data, which can be used later to pass data to your pages.